Is there a difference between "==" and "is" in Python?
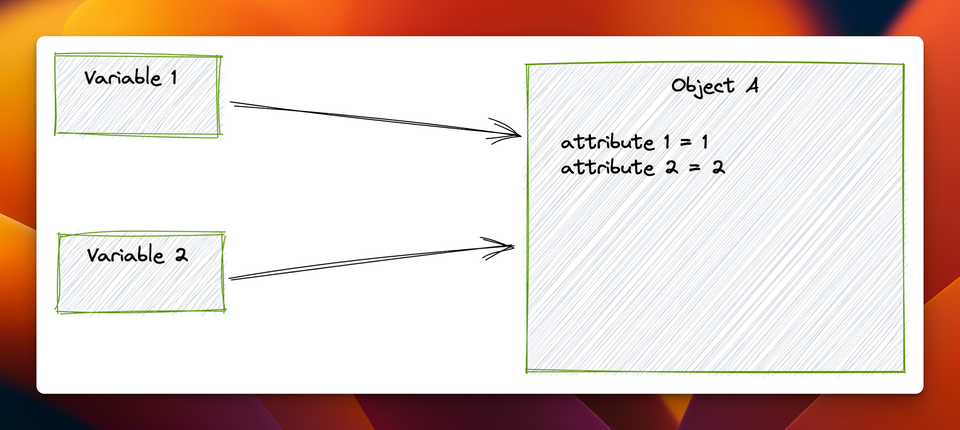
Are you wondering why sometimes is
and ==
are returning different results? Are you confused about when it is correct to use object identity is
for comparisons and when you should use the equals ==
operator?
Don't worry, I'll try to explain when you should use each one and the quirks around using them.
Well, there's a very specific difference. The rule of thumb would be to use is
for object reference equality and ==
for value equality. Let's see some examples to distinguished the different behaviour between the two.
Comparing integers
Let's try different examples in an interactive Python interpreter (ipython).
I am defining an integer and then trying both operators. First, the ==
operator returns True. The same holds for the is
operator. Although, the interpreter gives us a warning here the result is still True.
n = 3
n == 3
True
n is 3
# <>:1: SyntaxWarning: "is" with a literal. Did you mean "=="?
# <ipython-input-10-25443ad3dcce>:1: SyntaxWarning: "is" with a # literal. Did you mean "=="?
# n is 3
True
If the is
operator compares object references why the above example does not return False when we do n is 3
. This is because Python caches small integers which are integers between -5 and 256. Let's try the same example with bigger integers.
n = 257
n is 257
# <>:1: SyntaxWarning: "is" with a literal. Did you mean "=="?
# <ipython-input-26-92d0fb8e77b6>:1: SyntaxWarning: "is" with a# literal. Did you mean "=="?
# n is 257
False
Is clear now that is
could not be used when comparing integers or in general testing equality.
To put it differently is
is used for object identity. Therefore, it checks if objects refer to the same instance meaning the same address in memory. The ==
operator refers to equality meaning that two objects have the same value. For comparing objects using the ==
the __eq__(self)
method is used. If you create your own classes and objects you might want to overwrite the __eq__(self)
method to compare values between objects that are relevant to your business logic.
Let's visualise the concepts above
The first 2 images show two variables pointing to the same object. When comparing the two variables using the is
operator it will return True
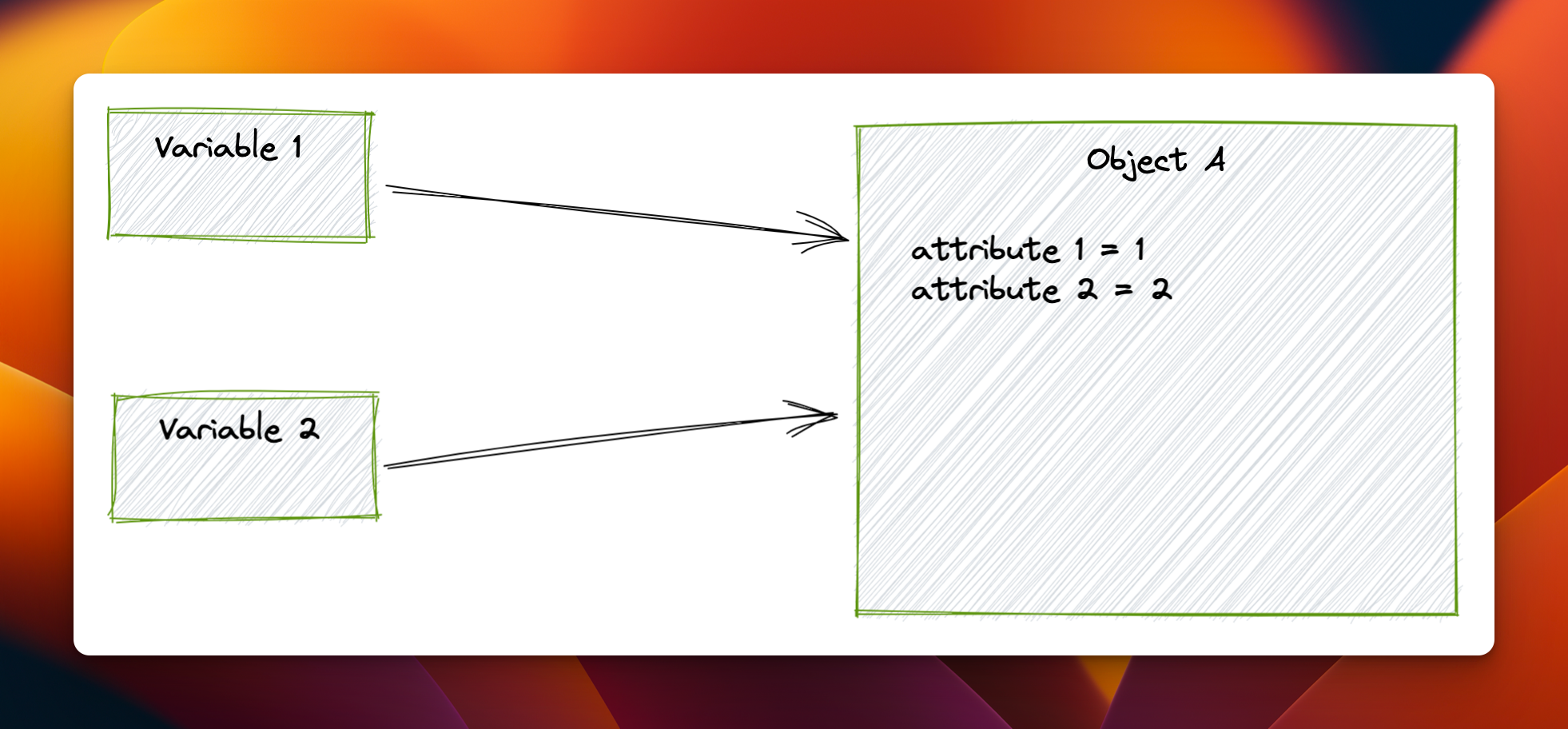
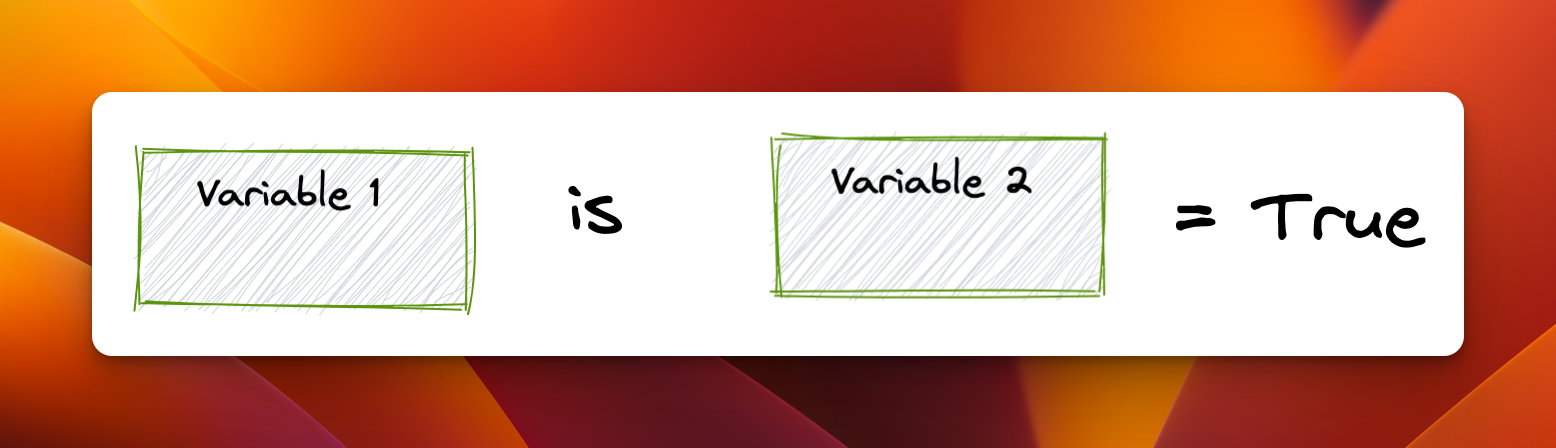
However, the next 2 pictures show when the results of is
and ==
operators will be different. In this example, variable 1 points to Object A and variable 2 points to Object B. This means that testing the two variables using the is
operator will return False
, since we have two different objects (different positions in memory) but if we compare the two objects using the ==
operator this will result to True.
NOTE: Here I've skipped an important detail which is the implementation of the __eq__(self)
method. But let's assume that the __eq__(self)
compares the values of attribute 1
and attribute 2
of the objects.
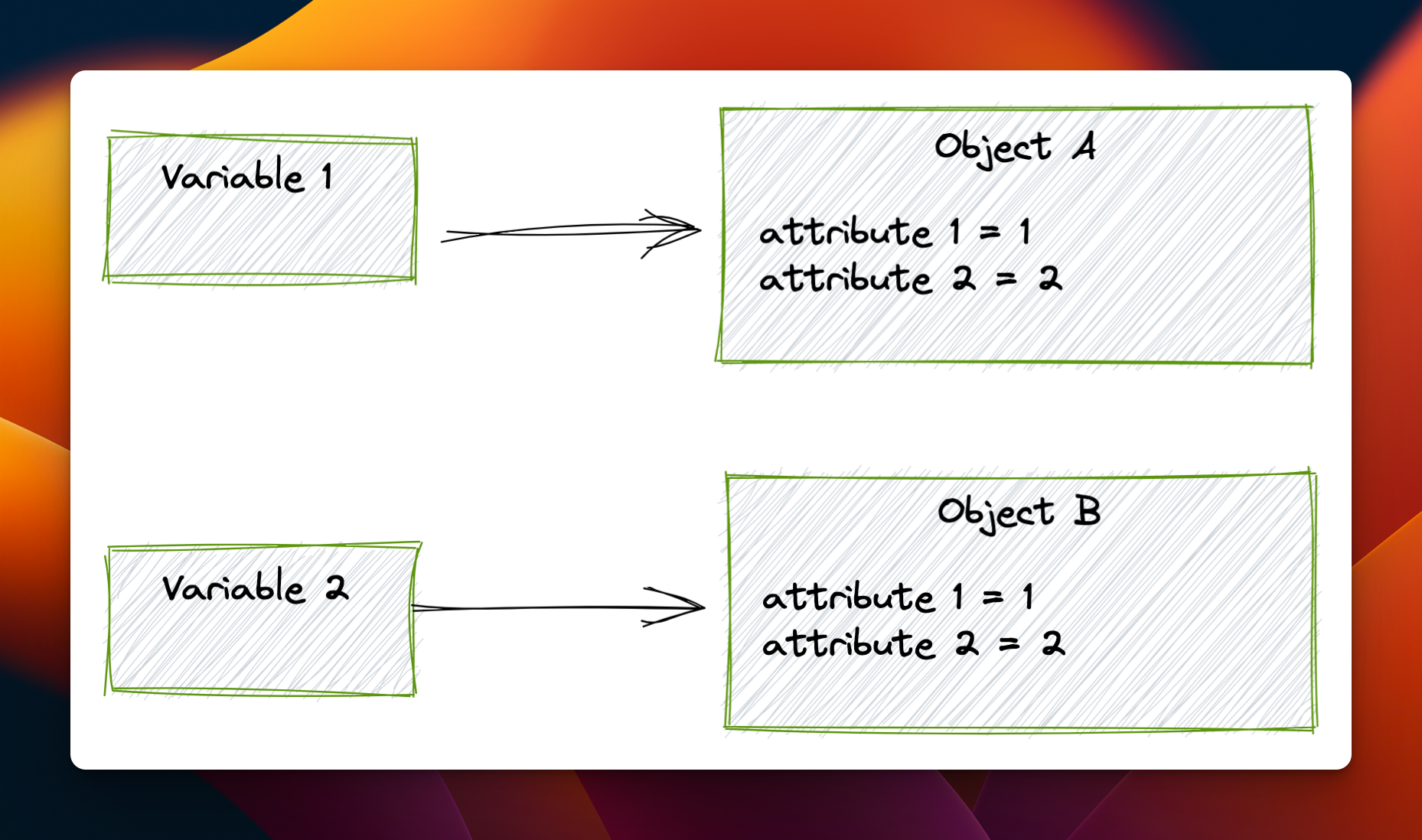
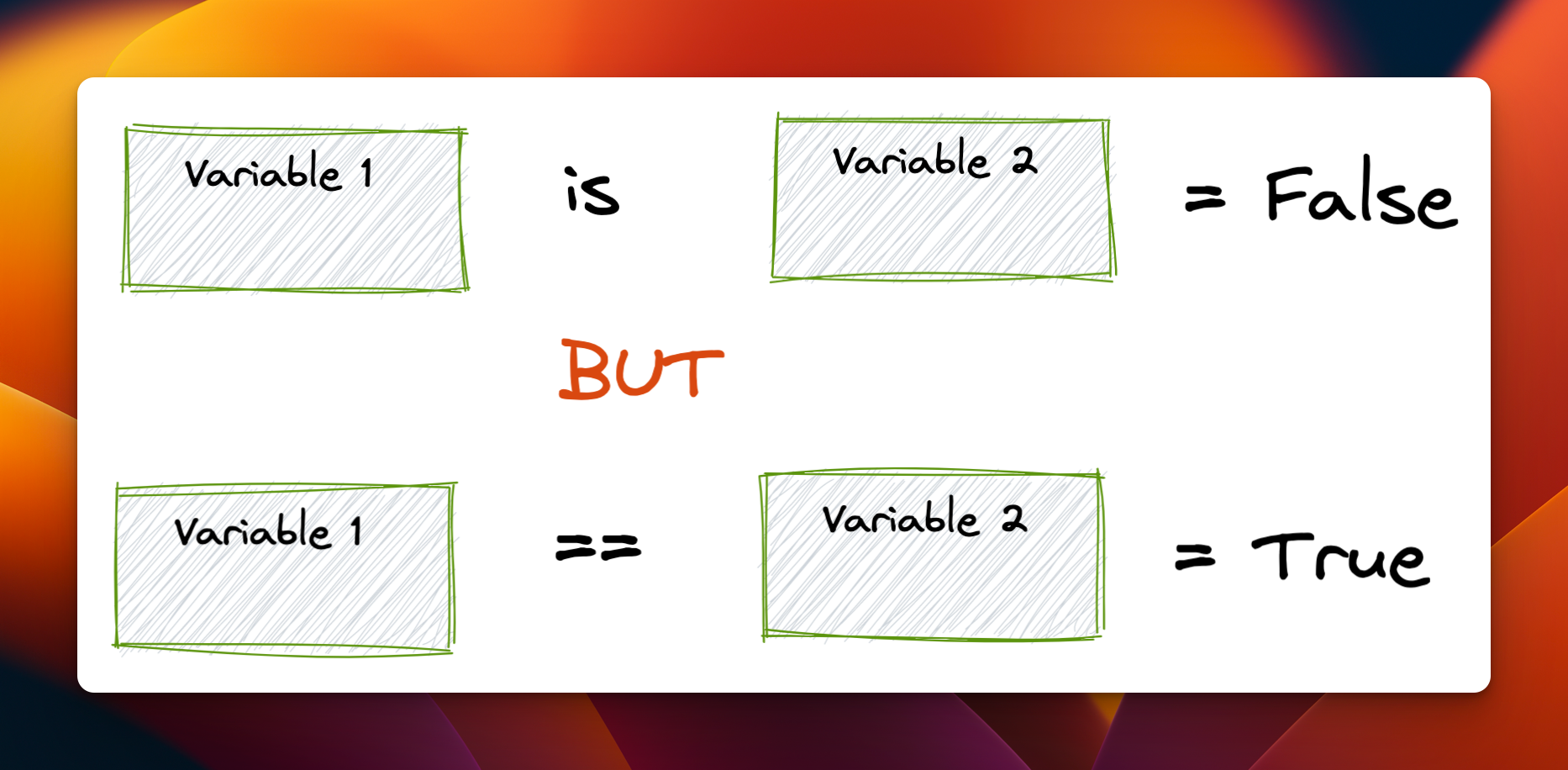
The end
Congratulations! You made it to the end.
If you like the content here, please consider subscribing. No spam ever!
If you have any comments or feedback feel free to reach me @costapiy
Member discussion