What is slicing in Python?
In Python, slicing is an operation of slicing a list. This means that it can extract some elements from a list using some preferred indexes. It allows you to select a range of elements from a list by creating a shallow copy of a list containing only those elements.
The easiest way to remember it is my_list[start:stop:step]
Different python slice combinations
All the parameters are optional. If a parameter is not set explicitly, Python will use a default value.
What are the default values for a python slice?
- For
start
the default value is0
. - For
:stop
the default value is the length of the list. - For
:step
the default value is1
Which values are included in the slice?
You might also wonder if the :stop
index will be included in the slice or not. The answer is no. The difference between start
and :stop
is that the element at index start
is included in the slice where the element at index :stop
is not.
elements[start:stop] # a slice of the array from the start element until the stop - 1 element
elements[start:] # a slice of the array from the start element until the end of the list.
elements[:stop] # a slice of the array from the start of the list until the stop - 1 element
elements[:] # a copy of the whole list
elements[start:stop:step] # a slice of the array from the start element until the stop - 1 element, by moving by step index each time
If we want to visualise it with an example:
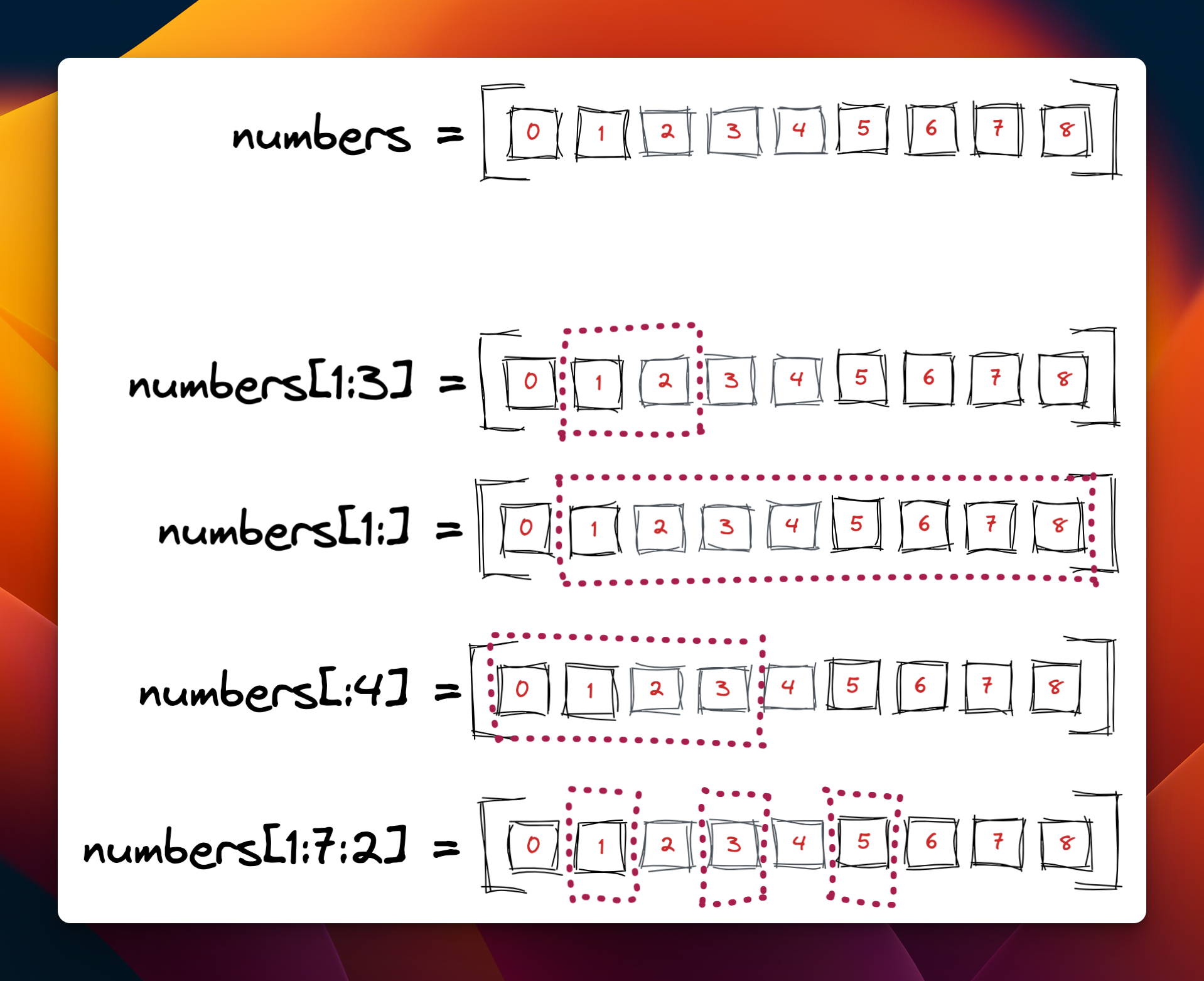
Python slice negative index
When using python slices you can also add negative indexes! What does this mean? Well, it means that lists in Python are a bit different than let's say arrays in other languages. The negative index is an index relative to the end of the list. The index -1
is the last element of the list. The index -2
is the second to last element and so on. Also, you can use a minus value for the step function. This means that the list is traversed in the opposite direction. There are more examples below to help you understand the quirks of using slices.
For example,
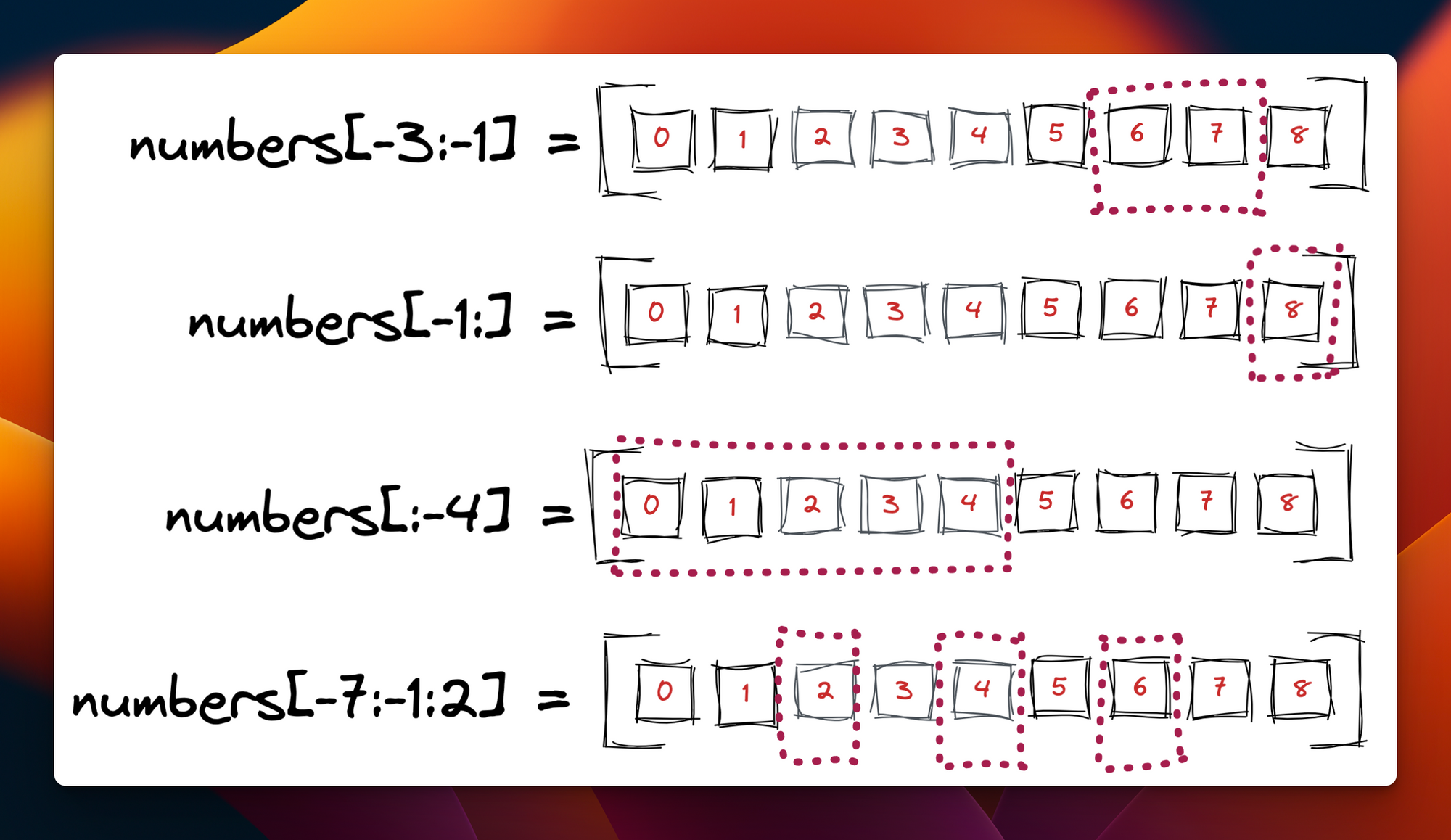
The slice()
function
In addition to the above you can also use the slice(start, stop, end)
function. This will create a slice object which then can be passed as a parameter to a list as shown in the example below:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8]
my_slice = slice(1, 3)
numbers[my_slice]
# [1, 2]
However, from my experience most people use the list[start, stop, step]
notation.
Code examples of Python slice
Here are more examples to help you completely understand how slices work.
Using positive indexes:
# list of numbers
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# returns the first five elements
print(numbers[:5])
[0, 1, 2, 3, 4]
# returns the last five elements
print(numbers[5:])
[5, 6, 7, 8, 9]
# returns the middle five elements
print(numbers[2:7])
[2, 3, 4, 5, 6]
# returns every other element
print(numbers[::2])
[0, 2, 4, 6, 8]
# returns every third element
print(numbers[::3])
[0, 3, 6, 9]
Using negative indexes:
# string of characters
chars = 'abcdefghijklmnopqrstuvwxyz'
# returns the last five characters
print(chars[-5:])
'vwxyz'
# returns the first five characters
print(chars[:-5])
'abcdefghijklmnopqrstu'
# returns the middle five characters
print(chars[11:-11])
'klmno'
# returns every other character
print(chars[::2])
'acegikmoqsuwy'
# returns every third character
print(chars[::3])
'adgjmpsvy'
And some more complex examples here:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Returns all elements from index 3 to the end of the list
numbers[3:]
[3, 4, 5, 6, 7, 8, 9]
# Returns all elements from the start of the list to index 6 (not including element at index 6)
numbers[:6]
[0, 1, 2, 3, 4, 5]
# Returns all elements from the start of the list to the end, skipping every other element
numbers[::2]
[0, 2, 4, 6, 8]
# Returns all elements from the end of the list to the start, in reverse order
numbers[::-1]
[9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
# Returns all elements from index 3 to index 6 (not including element at index 6), in reverse order
numbers[6:3:-1]
[6, 5, 4]
# Returns the list in reverse order
numbers[::-1]
[9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
I hope that this will help you understand Python slices once and for all.
The end
Congratulations! You made it to the end.
If you like the content here, please consider subscribing. No spam ever!
If you have any comments or feedback feel free to reach me @costapiy
Member discussion